This year's "What's new in Swift" included a plethora of improvements and new add-ons. Some of the news are:
- better diagnostic compiler error's and hence better debugging
- improved and faster code completion
- improved auto indentation
- improved integration
- improved handling of chained method calls and property accesses
- A standardized way to delegate a program's entry point with the
@main
attribute - Swift Numerics, an open source project bringing additional support for numeric functions to Swift
- Swift Argument Parser, build your command line tools with Swift
where
clauses on contextually generic declarations- Multi-Pattern
catch
clauses - Float16
But the list is much longer. In the following are some highlights for what is to come with Swift 5.3 and you can find a list of further reading and related videos at the bottom of the post.
Additional Cross Platform Support and Swift AWS Lambdas
To support the Swift ecosystem Apple has added additional cross-platform support and will officially support:
- All Apple platforms
- Ubuntu 16.04, 18.04, 20.04
- CentOS
- Amazon Linux 2
- Windows (coming from Swift 5.3)
This also opens up for official support for Swift AWS Lambdas. The AWSLambdaRuntime
package is based on SwiftNIO
and allows for both closure based Lambdas and eventloop Lambda functions. Below is an example of a closure based Lambda as presented in the talk:
import AWSLambdaRuntime
Lambda.run { (_, name: String, callback) in
callback(.success("Hello, \(name)!"))
}
Enum Improvements
This year we get two big improvements to the enum
type:
Synthesized Comparable
Conformance For enum
Types
With Swift 4.1 came synthesized, opt-in enum
conformance for Equatable
and Hashable
, however conformance to Comparable
was left out. From Swift 5.3 Comparable
will also be included, allowing for easy sorting by the order of declaration. The synthesized, opt-in conformace only comes for enum
s without raw values, without associated types or with comparable associated types.
enum ApplePlatform: Comparable {
case iOS
case ipadOS
case macOS
case tvOS
case watchOS
}
[ApplePlatform.watchOS, ApplePlatform.ipadOS, ApplePlatform.macOS].sorted()
// [ApplePlatform.ipadOS, ApplePlatform.macOS, ApplePlatform.watchOS]
Enum Cases As Protocol Witnesses
Another great improvement to enum
s in Swift is the possibility to allow for static protocol requirements to be witnessed by an enum
case. It introduces two rules:
- static, get-only protocol requirement can be witnessed by an enum case without an associated value
- static func requirement can be witnessed by an enum case with one or more associated values
protocol ErrorReportable {
static var notFound: Self { get }
static func invalid(searchTerm: String) -> Self
}
enum UserError: ErrorReportable {
case notFound
case invalid(searchTerm: String)
}
Multiple Trailing Closures
If you have been following along in the discussions on Swift forums, you may have come across the topic of multiple trailing closures. It has been thoroughly discussed and many people had their opinions on both the syntax and the final process.
Up until now it has only been possible to use a trailing closure if your method has one escaping closure. An example of that is one of the animate methods in UIKit
import UIKit
UIView.animate(withDuration: 0.3) {
self.view.alpha = 0
}
If we instead wanted to use the animate method that also allows us to define what should happen at completion we can't use trailing closures anymore
import UIKit
UIView.animate(
withDuration: 0.3,
animations: { self.view.alpha = 0 },
completion: { _ in
self.view.removeFromSuperview()
}
)
With Swift 5.3 any method can now have multiple trailing closures. The first closure will be without a label, while all remaining closures should be labeled. The animate method from before will now look like:
import UIKit
UIView.animate(withDuration: 0.3) {
self.view.alpha = 0
} completion: { _ in
self.view.removeFromSuperview()
}
Increased Availability of Implicit self
in Closures
Swift requires the explicit use of self
in escaping closures which capture it. This can lead to a lot of repetitive code. With implicit self
in closures in Swift 5.3, we can omit all self.
inside our closures. Instead we can add self
to the capture list of the closure. Using implicit self
will then update our animate method from before, so we only have to specify self
once in each closure.
import UIKit
UIView.animate(withDuration: 0.3) { [self] in
view.alpha = 0
} completion: { [self] _ in
view.removeFromSuperview()
}
Other WWDC Articles
Recommended WWDC Sessions
- What's new in Swift
- Explore logging in Swift
- Explore numeric computing in Swift
- Use Swift on AWS Lambda with Xcode
Resources and Further Reading
- Keypath expressions as functions (SE-0249)
- Callable values of user-defined nominal types (SE-0253)
- String initializer with access to uninitialized storage (SE-0263)
- Standard library preview package (SE-0264)
- Synthesized comparable conformance for enum types (SE-0266)
- Where Clauses on contextually generic declarations (SE-0267)
- Refined didSet semantics (SE-0268)
- Increased availability of implicit self in closures (SE-0269)
- Collection operations on noncontiguous elements (SE-0270)
- Multi-pattern catch clauses (SE-0276)
- Float16 (SE-0277)
- Multiple trailing closures (SE-0279)
- Enum cases as protocol witnesses (SE-0280)
- Type-based program entry points (SE-0281)
- Standard library preview
- Swift Numerics
- Swift Argument Parser
- SwiftLog
Article Photo - capture from What's new in Swift by Apple
Author
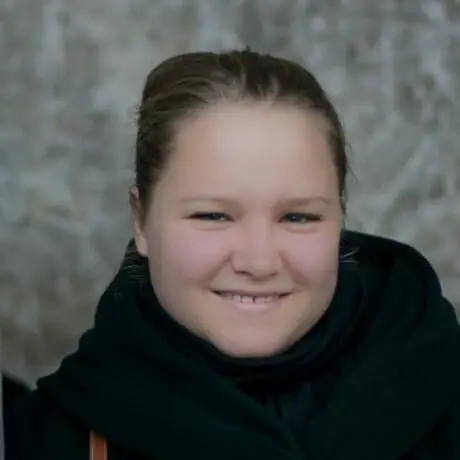
Heidi Puk Hermann
Vapor Developer